How to Program a Form with a Color transition Effect in Csharp
A program that changes the color of the form using 3 Timer
control object.
You only need 1 Label and 3 Timer
After adding the 3 timer, rename it to timer1 – timerR,
timer2 – timerG, timer3 – timerB. Set each Interval to 20 and Enabled to True.
Type the code to your code editor
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace ColorTransition
{
public partial class Form1 :
Form
{
public Form1()
{
InitializeComponent();
}
int r = 244;
int g = 65;
int b = 65;
private void
timerR_Tick(object sender, EventArgs e)
{
if (b >= 244)
{
r -= 1;
this.BackColor =
Color.FromArgb(r, g, b);
if (r <= 65)
{
timerR.Stop();
timerG.Start();
}
}
if (b <= 65)
{
r += 1;
this.BackColor =
Color.FromArgb(r, g, b);
if (r >= 244)
{
timerR.Stop();
timerG.Start();
}
}
}
private void
timerG_Tick(object sender, EventArgs e)
{
if (r <= 65)
{
g += 1;
this.BackColor =
Color.FromArgb(r, g, b);
if (g >= 244)
{
timerG.Stop();
timerB.Start();
}
}
if (r >= 244)
{
g -= 1;
this.BackColor =
Color.FromArgb(r, g, b);
if (g <= 65)
{
timerG.Stop();
timerB.Start();
}
}
}
private void
timerB_Tick(object sender, EventArgs e)
{
if (g <= 65)
{
b += 1;
this.BackColor =
Color.FromArgb(r, g, b);
if (b >= 244)
{
timerB.Stop();
timerR.Start();
}
}
if (g >= 244)
{
b -= 1;
this.BackColor =
Color.FromArgb(r, g, b);
if (b <=64)
{
timerB.Stop();
timerR.Start();
}
}
}
}
}
The output will change the form’s color.
How to Program a Form with a Color transition Effect in Csharp
Reviewed by code-dev
on
11:02 PM
Rating:
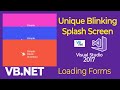
No comments: